The Power of Backtesting in Algorithmic Trading
Backtesting is an indispensable technique in the realm of algorithmic trading, enabling traders and developers to evaluate strategies and optimize performance before deploying them in live markets. By simulating trading activities using historical data, backtesting offers valuable insights into a trading bot’s potential effectiveness. This process minimizes risks, reduces uncertainties, and helps in creating more robust and profitable trading algorithms.
At the heart of trading bot backtesting samples with Python lies the ability to harness the power of this versatile programming language. Python’s extensive libraries, such as Backtrader, Zipline, and Catalyst, provide developers with the necessary tools to design, implement, and analyze backtesting samples efficiently. These libraries simplify the process of data handling, strategy development, and performance evaluation, making Python an ideal choice for trading bot backtesting.
When embarking on a trading bot development journey, it is crucial to select appropriate backtesting samples. Factors to consider include data quality, timeframes, and market conditions. High-quality data, relevant timeframes, and realistic market scenarios are essential for generating reliable backtesting results. By carefully choosing and preparing backtesting samples, developers can create more accurate simulations, thereby increasing the likelihood of successful trading bot performance in live markets.

Selecting the Right Backtesting Samples
Choosing appropriate backtesting samples is a critical step in trading bot development. High-quality backtesting samples enable developers to create realistic simulations of trading activities, ensuring that the trading bot can effectively navigate various market conditions. By carefully selecting and preparing backtesting samples, developers can minimize risks, optimize performance, and increase the likelihood of success in live markets.
When choosing backtesting samples, several factors must be taken into account. First and foremost, data quality is paramount. High-quality data should be accurate, consistent, and free from errors or inconsistencies. Utilizing reliable data sources ensures that the backtesting results are representative of real-world market scenarios, thereby increasing the confidence in the trading bot’s performance.
Timeframes are another essential factor to consider when selecting backtesting samples. The chosen timeframe should be relevant to the trading strategy being evaluated. For instance, intraday traders may prefer shorter timeframes, while long-term investors might opt for longer timeframes. Ensuring that the timeframe aligns with the trading strategy helps create more accurate simulations and reduces the risk of misleading backtesting results.
Market conditions also play a crucial role in backtesting sample selection. Developers should consider various market scenarios, such as bull markets, bear markets, and sideways markets, when preparing backtesting samples. Including diverse market conditions in the backtesting process ensures that the trading bot can adapt to different market environments, thereby increasing its robustness and effectiveness.
In summary, selecting appropriate backtesting samples is a critical aspect of trading bot development. By focusing on data quality, timeframes, and market conditions, developers can create realistic simulations that accurately reflect the trading bot’s potential performance in live markets. High-quality backtesting samples, combined with powerful tools like Python and its libraries, enable developers to build, evaluate, and optimize trading bots with confidence.

Harnessing Python for Trading Bot Backtesting
Python has emerged as a powerful and popular tool for trading bot backtesting, offering developers a wide range of libraries and frameworks to streamline the development process. With its versatile syntax, extensive community support, and a vast array of data analysis tools, Python is an ideal choice for building and evaluating trading bots.
Several Python libraries and frameworks are specifically designed for trading bot backtesting. Among the most popular are Backtrader, Zipline, and Catalyst. These tools provide developers with pre-built functionality for data handling, strategy implementation, and performance evaluation, enabling them to create sophisticated trading bots with relative ease.
Backtrader is a feature-rich, open-source Python framework for backtesting and trading. It supports multiple data feeds, offers advanced order management, and allows for custom indicators and strategies. Backtrader’s flexibility and extensive documentation make it an excellent choice for both beginners and experienced developers.
Zipline is another open-source Python library for backtesting algorithmic trading strategies. Developed by Quantopian, Zipline is designed for high-performance backtesting and supports both event-driven and time-based strategies. Its simple syntax and integration with popular data sources, such as Quandl and Yahoo Finance, make it an attractive option for developers seeking a user-friendly backtesting solution.
Catalyst is a high-performance, open-source backtesting and live trading framework for Python and CuPy. Built for speed and scalability, Catalyst supports both equities and cryptocurrency trading, offering advanced features such as custom data handlers, multi-threading, and real-time data processing. Catalyst is particularly well-suited for developers working with large datasets or implementing complex trading strategies.
In conclusion, Python offers a wealth of libraries and frameworks for trading bot backtesting, enabling developers to create robust and sophisticated trading bots. By leveraging the power of tools like Backtrader, Zipline, and Catalyst, developers can streamline the backtesting process, evaluate strategies, and optimize performance, ultimately increasing the likelihood of success in live markets.

How to Implement Backtesting Samples with Python
To implement backtesting samples using Python, follow these steps to ensure a smooth and efficient development process:
- Choose a Python library or framework: Select a suitable Python library or framework for your project, such as Backtrader, Zipline, or Catalyst. Each tool offers unique features and capabilities, so consider your specific requirements and choose the one that best aligns with your needs.
- Prepare your data: High-quality data is crucial for accurate backtesting. Ensure that your data is clean, relevant, and well-structured. Preprocess the data as needed, including removing missing values, handling outliers, and normalizing the data. Store the data in a format compatible with your chosen Python library or framework.
- Define your strategy: Clearly outline your trading strategy, including entry and exit rules, position sizing, and risk management techniques. Implement the strategy using the syntax and functions provided by your chosen Python library or framework.
- Configure backtesting parameters: Set up the backtesting environment by specifying the timeframe, data frequency, and other relevant parameters. This step may involve defining the start and end dates for the backtesting period, selecting a data feed, and configuring any necessary data handlers.
- Run the backtest: Execute the backtest using your Python library or framework. Monitor the progress and ensure that there are no errors or exceptions. Allow the backtest to run until completion, generating a set of results and performance metrics.
- Analyze the results: Evaluate the backtesting results to determine the effectiveness of your trading strategy. This step may involve calculating key performance indicators (KPIs), such as profitability, risk, and drawdowns, and visualizing the results using charts and graphs.
- Iterate and refine: Based on your analysis, refine your strategy, adjust parameters, and iterate the backtesting process. Continuously improve your trading bot by incorporating new data, market conditions, and insights to optimize performance.
Here’s a simple example of a Python function that implements a basic moving average crossover strategy using the Backtrader library:
import backtrader as bt class MovingAverageCrossover(bt.Strategy):
params = (('fast', 10), ('slow', 30),)
def __init__(self):
self.sma_fast = bt.indicators.SimpleMovingAverage(self.data, period=self.params.fast)
self.sma_slow = bt.indicators.SimpleMovingAverage(self.data, period=self.params.slow)
def next(self):
if self.sma_fast[0] > self.sma_slow[0] and self.sma_fast[-1] < self.sma_slow[-1]:
self.buy()
elif self.sma_fast[0] < self.sma_slow[0] and self.sma_fast[-1] > self.sma_slow[-1]:
self.sell()
This example demonstrates how to create a custom strategy class, define indicators, and implement entry and exit rules using the Backtrader library.
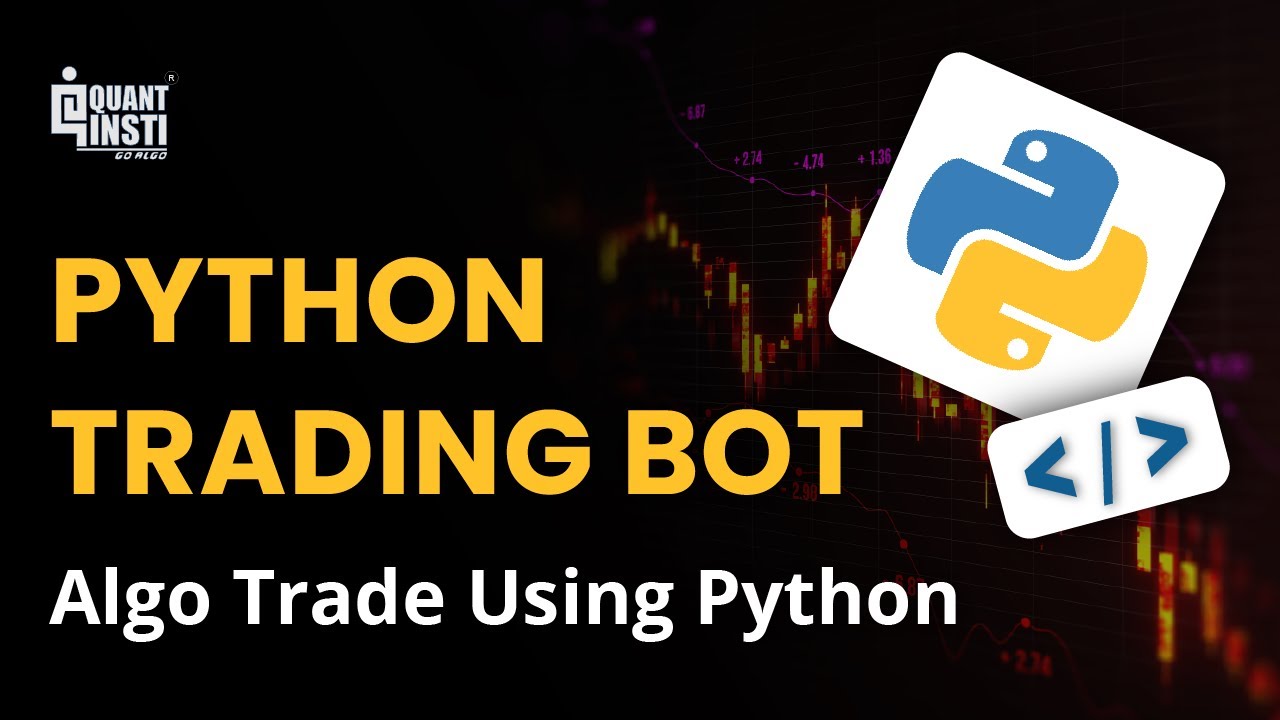
Analyzing Backtesting Results for Improved Trading Bot Performance
Once you have implemented backtesting samples using Python, the next critical step is analyzing the results to optimize trading bot performance. This process involves evaluating key performance indicators (KPIs) that shed light on the profitability, risk, and drawdowns of your strategy. By understanding these metrics, you can refine your strategy, address potential issues, and improve overall performance.
Profitability Metrics
Profitability metrics help you assess the financial gains of your trading strategy. Some essential profitability KPIs include:
- Total Net Profit: The difference between the total gains and total losses of your strategy.
- Profit Factor: The ratio of total gains to total losses, indicating the efficiency of your strategy.
- Return on Investment (ROI): The percentage return on your initial investment, calculated as (Net Profit / Initial Investment) x 100.
- Compounded Annual Growth Rate (CAGR): The annual growth rate of your investment, accounting for compounding effects.
Risk Metrics
Risk metrics help you understand the potential losses and volatility associated with your trading strategy. Some crucial risk KPIs are:
- Maximum Drawdown: The largest peak-to-valley loss experienced during the backtesting period.
- Calmar Ratio: The ratio of annualized net profit to maximum drawdown, indicating the risk-adjusted performance of your strategy.
- Sortino Ratio: A variation of the Calmar Ratio that considers only downside volatility, providing a more nuanced view of risk.
- Value at Risk (VaR): The minimum loss expected over a specified time horizon with a given confidence level.
Visualizing Results
Visualizing backtesting results can provide valuable insights into your trading strategy’s performance. Use charts and graphs to display KPIs, such as:
- Equity Curve: A graph showing the evolution of your trading account’s value over time.
- Drawdown Distribution: A histogram displaying the frequency and magnitude of drawdowns.
- Heatmaps: A visual representation of the correlation between different assets or strategies.
By analyzing backtesting results, you can identify areas for improvement, refine your trading strategy, and optimize performance. Continuously monitor and update your KPIs to ensure your trading bot remains effective and adaptive to changing market conditions.

Selecting the Best Trading Bot Backtesting Tools in Python
When it comes to trading bot backtesting samples with Python, choosing the right libraries and frameworks is crucial for success. Various options are available, each with its unique strengths and weaknesses. By understanding these differences, you can make informed decisions and select the best tools for your specific use case.
Backtrader
Backtrader is a versatile and feature-rich backtesting library for trading bots. Its primary advantages include:
- Flexibility: Backtrader supports multiple data feeds, making it compatible with various data sources.
- Extensibility: Users can create custom indicators, strategies, and analyzers with ease.
- Simplicity: Backtrader’s straightforward syntax and comprehensive documentation make it accessible to both beginners and experienced developers.
Zipline
Zipline is a popular open-source backtesting library developed by Quantopian. Key features of Zipline include:
- Efficiency: Zipline is optimized for performance, allowing for rapid backtesting of trading strategies.
- Integration: Zipline integrates seamlessly with Quantopian’s research environment and IPython notebooks.
- Community: Zipline boasts a large and active community, ensuring regular updates and improvements.
Catalyst
Catalyst is a high-performance backtesting library designed for building and deploying trading algorithms. Its main benefits are:
- Scalability: Catalyst can handle large datasets and complex trading strategies, making it suitable for professional traders and institutions.
- Modularity: Catalyst’s modular design allows for easy customization and integration with other tools and platforms.
- Live Trading: Catalyst supports live trading, enabling users to deploy and manage trading bots in real-time.
Recommendations
The best trading bot backtesting tool for you depends on your specific needs and expertise. For beginners, Backtrader’s simplicity and flexibility make it an excellent starting point. Zipline is a solid choice for those interested in using Quantopian’s research environment, while Catalyst is ideal for professionals seeking a high-performance, scalable solution.
When selecting a backtesting tool, consider factors such as data compatibility, customization options, and community support. By carefully evaluating these aspects, you can ensure that your chosen library or framework meets your requirements and helps you build a successful trading bot.

Strategies for Overcoming Common Backtesting Challenges
Trading bot backtesting samples with Python offers numerous benefits, but it also comes with its own set of challenges. By understanding these issues and implementing strategies to address them, you can improve backtesting accuracy and build more effective trading bots.
Overfitting
Overfitting occurs when a trading strategy is excessively tailored to historical data, resulting in poor performance when applied to new, unseen data. To prevent overfitting, consider the following strategies:
- Cross-validation: Divide your data into multiple subsets for training and testing. This process helps ensure that your strategy can generalize well to new data.
- Simplification: Opt for simpler models and trading rules, as complex strategies are more likely to overfit.
- Regularization: Implement regularization techniques, such as L1 or L2 regularization, to reduce overfitting in your models.
Lookahead Bias
Lookahead bias occurs when a backtesting sample uses information not available at the time of the decision, leading to unrealistic performance expectations. To avoid lookahead bias, follow these guidelines:
- In-sample and out-of-sample data: Split your data into in-sample and out-of-sample sets. Use only the in-sample data for training and reserve the out-of-sample data for testing.
- Use of future information: Ensure that your strategy does not use any information not yet available at the time of the decision.
- Realistic data: Utilize real-world data, including weekends, holidays, and other factors that can impact trading decisions.
Curve Fitting
Curve fitting is the process of adjusting a strategy’s parameters to fit historical data, leading to inflated performance metrics. To minimize curve fitting, consider the following:
- Parameter optimization: Use techniques like grid search or random search to find optimal parameters, rather than manually adjusting them.
- Walk-forward optimization: Implement walk-forward optimization, where you train your model on past data, test it on future data, and then retrain it with updated data.
- Statistical significance: Perform statistical tests to ensure that your strategy’s performance improvements are statistically significant and not merely the result of random chance.
By addressing these common challenges, you can improve the accuracy and reliability of your trading bot backtesting samples with Python. This, in turn, will help you build more effective trading bots capable of delivering consistent results in various market conditions.

Continuous Improvement: Updating and Refining Backtesting Samples
Trading bot backtesting samples with Python are essential for evaluating, minimizing risks, and optimizing the performance of algorithmic trading strategies. However, the process of backtesting should not be a one-time event. Instead, it should be an ongoing effort to ensure that your trading bot remains effective and up-to-date in various market conditions.
To maintain the relevance and accuracy of your trading bot backtesting samples with Python, consider the following practices:
- Regular updates: Periodically update your backtesting samples with new data to ensure that your trading bot remains adaptable to changing market conditions.
- Market analysis: Stay informed about recent market trends, news, and events. Incorporate this knowledge into your backtesting samples to improve their accuracy and applicability.
- Strategy evaluation: Continuously evaluate your trading strategy’s performance based on key performance indicators (KPIs) such as profitability, risk, and drawdowns. Adjust your strategy as needed to optimize its performance.
- Iterative improvement: Implement an iterative improvement process, where you refine your backtesting samples based on the insights gained from your analysis. This process may involve adjusting parameters, modifying trading rules, or even changing the underlying model.
By following these practices, you can ensure that your trading bot backtesting samples with Python remain relevant and effective in various market conditions. Continuous improvement not only helps you build a better trading bot but also enables you to stay ahead of the competition and maximize your profitability in the world of algorithmic trading.